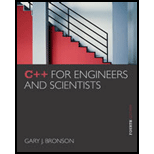
(a)
To write a function that calculate the area of a circle. It call another function to get the radius of the circle. The circumference of the circle is given.
(a)

Explanation of Solution
The name of the function is area().
This function has one parameters.
The parameter will accept afloating number to get the circumference (c) of a circle.
It call another function named radius () to get the radius (r) of the circle.
This function has one parameters.
The parameter will accept a floating number to get the circumference (c) of a circle.
The formula to calculate the radius of the circle is c / 2π.
The formula to calculate the area of the circle is πr2.
The function definition is:
float radius (float circumference) { //variable declaration float r; //calculate radius of the circle r = c / (2 * PI); //return radius return r; } //function to calculate area void area (float c) { //variable declaration float a, r; //get the radius of the circle by calling the function r = radius(c); //calculate area of the circle a = PI * pow (r, 2); //display radius of the circle cout<<"The radius of the circle is: "<< r <<endl; //display area of the circle cout<<"The area of the circle is: "<< a; }
(b)
- c,r,andavariables are used in the program.
- area () function is include in the program to calculate and display the area of the circle.
- radius () function is include in the program tocalculate and return the radius of the circle.
Program Description: The main purpose of the program is to prompt the user to enter the circumference of a circle to calculate the radius and the area of that circle. Include the function named area() in the main program. Call the function from the main () function with the value of the circumference of a circle.
(b)

Explanation of Solution
Program:
//including essential header file #include <iostream> #include <cmath> //define the value of PI (p) #define PI 3.14 //using standard namespace usingnamespace std; //function to calculate radius float radius (float circumference) { //variable declaration float r; //calculate radius of the circle r = circumference / (2 * PI); //return radius return r; } //function to calculate area void area (float c) { //variable declaration float a, r; //get the radius of the circle by calling the function r = radius(c); //calculate area of the circle a = PI * pow (r, 2); //display radius of the circle cout<<"The radius of the circle is: "<< r <<endl; //display area of the circle cout<<"The area of the circle is: "<< a; } //main function intmain() { //variable declaration float c; //prompt the user to enter the circumference of the circle cout<<"Enter the circumference of the circle: "; //get the circumference from the user cin>> c; //call the function area (c); return 0; }
Explanation: In the above code, themain() function is to prompt the user to enter the circumference of a circle. The user-entered circumference is stored in the variable named c. Then the main()function call a function named area()with the value of the circumference to calculate and display the radius and area of the circle. The function named area()has one parameter named c. The parameter named c stores the circumference of a circle passed by the main () function. The function namedarea()called another function to get the radius of the circle. Call the function named radius () with the circumference of a circle. The function named radius ()Calculate the radius of the circle by the given formula and return the radius of the circle.The function namedarea() calculates the area of the circle by the given formula and display the radius and area of the circle.
Sample Output:
Want to see more full solutions like this?
Chapter 6 Solutions
C++ for Engineers and Scientists
- (Wattan Corporation) is an Internet service provider that charges customers a flat rate of $7.99 for up to 10 hours of connection time. Additional hours or partial hours are charged at $1.99 each. Write a function charges that computes the total charge for a customer based on the number of hours of connection time used in a month. The function should also calculate the average cost per hour of the time used (rounded to the nearest 0.01), so use two output parameters to send back these results. You should write a second function round_money that takes a real number as an input argument and returns as the function value the number rounded to two decimal places. Write a main function that takes data from an input file usage.txt and produces an output file charges.txt. The data file format is as follows: Line 1: current month and year as two integers Other lines: customer number (a five-digit number) and number of hours used Here is a sample data file and the corresponding output file:…arrow_forward(python) Based on the following function definition, which answer is a proper function call. (select all correct answers) def greet(name, msg="Hello"): print(msg + ", " + name) a. greet("Seth") b. greet("Seth", "Hello") c. greet() d. greet(msg="Hello", "Seth") e. greet(msg="Hello", name="Seth")arrow_forward(a) Write a Python function that evaluates the following mathematical function. Note: x is a float and n is a natural number. f(z.n) = [(1+z)ex (1+ex (1+exx (1+²) nENarrow_forward
- (C PROGRAMMING ONLY) 7. Comparing Charactersby CodeChum Admin Most, if not all things have numeric values. That goes for characters too. In order to find out which character has a higher value, we have to compare them with each other and display the value of the higher character. Instructions: In the code editor, you are provided with a main() function that asks the user for 2 characters, passes these characters to a function call of the getHigherValue() function, and then prints out the ASCII value of the higher character.Your task is to implement the getHigherValue function which has the following details:Return type - intName - getHigherValueParameters - 2 characters to be comparedDescription - the ASCII value of the higher character.Hint: Comparing characters in C is just like comparing integers.DO NOT EDIT ANYTHING IN THE MAINInput 1. First character 2. Second character Output Enter first character: aEnter second character: hResult value = 104arrow_forward(C PROGRAMMING ONLY) DO NOT EDIT THE MAIN 7. Special Exchanging Giftsby CodeChum Admin It's Christmas time and we're having this exchanging gifts activity! I want to surprise the receiver of my gift. After he/she receives my gift, I will add an additional P100 bill! Instructions: In the code editor, you are provided with a main() function that asks the user for 2 integers. Then, a function call to a function named, exchangeGift() is made and the addresses of the 2 integers are passed.This exchangeGift() function hasn't been implemented yet so this is your task. This has the following description:Return type - voidName - exchangeGiftParameters - 2 addresses of 2 integersDescription - swaps the value of the 2 integers and then adds 100 to the 2nd integer (or 2nd parameter)DO NOT EDIT THE MAINInput 1. First integer 2. Second integer Output Enter integer 1: 10Enter integer 2: 2020 110arrow_forward(Use Python) The function course_average should calculate and return the average of the three values pass to it. The function main should ask the user to enter three grades and then pass these values to the course_average function. This function should also display a message to the user in the format below. For example, if the user entered 100, 90 and 95 the message would be:The average of 100 , 90 and 95 is 95arrow_forward
- (C PROGRAMMING ONLY) 4. Special Exchanging Giftsby CodeChum Admin It's Christmas time and we're having this exchanging gifts activity! I want to surprise the receiver of my gift. After he/she receives my gift, I will add an additional P100 bill! Instructions: In the code editor, you are provided with a main() function that asks the user for 2 integers. Then, a function call to a function named, exchangeGift() is made and the addresses of the 2 integers are passed.This exchangeGift() function hasn't been implemented yet so this is your task. This has the following description:Return type - voidName - exchangeGiftParameters - 2 addresses of 2 integersDescription - swaps the value of the 2 integers and then adds 100 to the 2nd integer (or 2nd parameter)DO NOT EDIT THE MAINInput 1. First integer 2. Second integer Output Enter·integer·1:·10Enter·integer·2:·2020·110arrow_forward(Apartment problem) A real estate office handles, say, 50 apartment units. When the rent is, say, $600 per month, all the units are occupied. However, for each, say, $40 increase in rent, one unit becomes vacant. Moreover, each occupied unit requires an average of $27 per month for maintenance. How many units should be rented to maximize the profit? Write a program in C++ that prompts the user to enter: The total number of units. The rent to occupy all the units. Amount to maintain a rented unit. The increase in rent that results in a vacant unit. The program then outputs: The number of units to be rented to maximize the profit The maximum profit Since your program handles currency, make sure to use a data type that can store decimals with a decimal precision of 2.arrow_forward(C PROGRAMMING ONLY) DO NOT EDIT THE MAIN FUNCTION 4. Simplicity is Beautyby CodeChum Admin In life, simplicity is beauty. Let's try creating something so simple. Let's create a function that accepts the address of an integer and prints n number of asterisks out of it. For example, if the value of the address passed is 5, then the output would be five asterisks in one line. Instructions: In the code editor, you are provided with a main() function that asks the user for an integer and passes the address of this integer to a function call of the simple() function.This simple() function hasn't been implemented yet so your task is just that, to implement it. This has the following description:Return type - voidName - simpleParameter - address of an integerDescription - prints a line of asterisksDO NOT EDIT THE MAIN FUNCTIONInput 1. An integer Output Enter n: 5*****arrow_forward
- (python) Write a function with name sqr that takes one argument, x. Write this function so that it computes the value x2, and returns this value. Ensure that no other output is given.arrow_forward(C PROGRAMMING ONLY) 2. Weird Treasure Chestby CodeChum Admin I'm now in the middle of the jungle when I saw this weird treasure chest. It seems that if I properly place the address of a certain number, I get a very weird result! Instructions: In the code editor, you are provided with a treasureChestMagic() function which has the following description:Return type - voidName - treasureChestMagicParameters - an address of an integerDescription - updates the value of a certain integer randomlyYou do not have to worry about how the treasureChestMagic() function works. All you have to do is ask the user for an integer and then call the treasureChestMagic() function, passing the address of that integer you just asked.Finally, print the updated value of the integer inputted by the user.Input 1. An integer Output Enter n: 10Treasure Chest Magic Result = 13arrow_forward(C++)Write a program that will allow a student to compute for his equivalent semestral grade. The program should ask the user should input his/her first name, last name, middle initial, and student number. After, inputting everything above, the output will clear the current screen and a new screen should open. A sample program that would clear a screen is included below for your reference. On the new screen, the user will then be greeted "Welcome, <firstname>! (i.e. Welcome, Louie!)" The program will then ask for the prelim grade, midterm grade and final grade of the student. The program should only accept grades 100 and below. After getting the three grades, the program should compute for the semestral grade. The computation of the semestral grade is as follows: 30%*PrelimGrade + 30%*midtermgrade + 40%finalgrade After inputting all the grades, the screen should clear again and will proceed to another screen. The new screen should output should output the following (this is…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
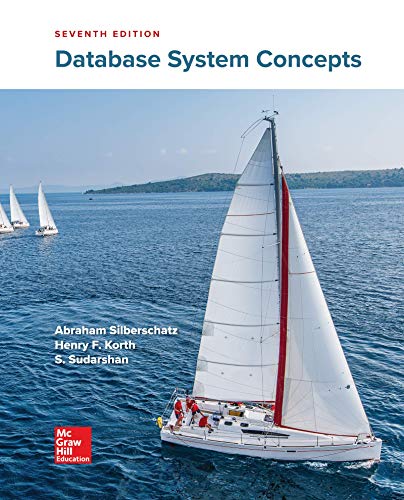
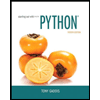
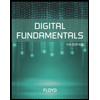
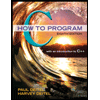
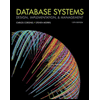
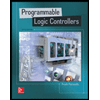