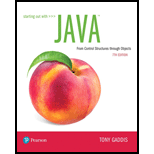
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 8, Problem 8PC
Parking Ticket Simulator
For this assignment you will design a set of classes that work together to simulate a police officer issuing a parking ticket. You should design the following classes:
- The ParkedCar Class: This class should simulate a parked car. The class’s responsibilities are as follows:
- To know the car’s make, model, color, license number, and the number of minutes that the car has been parked.
- The ParkingMeter Class: This class should simulate a parking meter. The class’s only responsibility is as follows:
- To know the number of minutes of parking time that has been purchased.
- The ParkingTicket Class: This class should simulate a parking ticker. The class’s responsibilities are as follows:
- To report the make, model, color, and license number of the illegally parked car
- To report the amount of the fine, which is $25 for the first hour or part of an hour that the car is illegally parked, plus $10 for every additional hour or part of an hour that the car is illegally parked
- To report the name and badge number of the police officer issuing the ticket
- The PoliceOfficer Class: This class should simulate a police officer inspecting parked cars. The class’s responsibilities are as follows:
- To know the police officer’s name and badge number
- To examine a ParkedCar object and a ParkingMeter object, and determine whether the car’s time has expired
- To issue a parking ticket (generate a ParkingTicket object) if the car’s time has expired
Write a program that demonstrates how these classes collaborate.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Dice Rolling Class
In this problem, you will need to create a program that simulates rolling dice. To start this project, you will first need to define the properties and behaviors of a single die that can be reused multiple times in your future code. This will be done by creating a Dice class.
Create a Dice class that contains the following members:
Two private integer variables to store the minimum and maximum roll possible.
Two constructors that initialize the data members that store the min/max possible values of rolls.
a constructor with default min/max values.
a constructor that takes 2 input arguments corresponding to the min and max roll values
Create a roll() function that returns a random number that is uniformly distributed between the minimum and maximum possible roll values.
Create a small test program that asks the user to give a minValue and maxValue for a die, construct a single object of the Dice class with the constructor that initializes the min and max…
Assignment:The BankAccount class models an account of a customer. A BankAccount has the followinginstance variables: A unique account id sequentially assigned when the Bank Account is created. A balance which represents the amount of money in the account A date created which is the date on which the account is created.The following methods are defined in the BankAccount class: Withdraw – subtract money from the balance Deposit – add money to the balance Inquiry on:o Balanceo Account ido Date created
Assignment 10: Chapter 10
Complete Programming Challenge 10 at the end of chapter 10
10. Ship, CruiseShip, and CargoShip Classes Design a Ship class that the following members: A field for the name of the ship (a string) - A
field for the year that the ship was built (a string). A constructor and appropriate accessors and mutators. A toString method that displays
the ship's name and the year it was built.
Design a CruiseShip class that extends the Ship class. The CruiseShip class should have the following members: A field for the maximum
number of passengers (an int). A constructor and appropriate accessors and mutators. A toString method that overrides the toString
method in the base class. The CruiseShip class's toString method should display only the ship's name and the maximum number of
passengers.
Design a CargoShip class that extends the Ship class. The CargoShip class should have the following members: A field for the cargo
capacity in tonnage (an int). A constructor and…
Chapter 8 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 8.1 - What is the difference between an instance field...Ch. 8.1 - Prob. 8.2CPCh. 8.1 - Describe the limitation of static methods.Ch. 8.8 - Prob. 8.4CPCh. 8.9 - Look at the following statement, which declares an...Ch. 8.9 - Assume that the following enumerated data type has...Ch. 8.9 - Prob. 8.7CPCh. 8 - This type of method cannot access any non-static...Ch. 8 - Prob. 2MCCh. 8 - If you write this method for a class, Java will...
Ch. 8 - Making an instance of one class a field in another...Ch. 8 - This is the name of a reference variable that is...Ch. 8 - This enum method returns the position of an enum...Ch. 8 - Assuming the following declaration exists: enum...Ch. 8 - You cannot use the fully qualified name of an enum...Ch. 8 - The Java Virtual Machine periodically performs...Ch. 8 - If a class has this method, it is called...Ch. 8 - CRC stands for a. Class, Return value, Composition...Ch. 8 - True or False: A static member method may refer to...Ch. 8 - True or False: All static member variables are...Ch. 8 - Prob. 14TFCh. 8 - Prob. 15TFCh. 8 - Prob. 16TFCh. 8 - True or False: Enumerated data types are actually...Ch. 8 - True or False: enum constants have a toString...Ch. 8 - public class MyClass { private int x; private...Ch. 8 - Assume the following declaration exists : enum...Ch. 8 - Consider the following class declaration: public...Ch. 8 - Consider the following class declaration: public...Ch. 8 - A pet store sells dogs, cats, birds, and hamsters....Ch. 8 - Prob. 1SACh. 8 - Prob. 2SACh. 8 - Prob. 3SACh. 8 - Even if you do not write an equals method for a...Ch. 8 - A has a relationship can exist between classes....Ch. 8 - Prob. 6SACh. 8 - Is it advisable or not advisable to write a method...Ch. 8 - Prob. 8SACh. 8 - Look at the following declaration: enum Color {...Ch. 8 - Assuming the following enum declaration exists:...Ch. 8 - Under what circumstances does an object become a...Ch. 8 - Area Class Write a class that has three overloaded...Ch. 8 - BankAccount Class Copy Constructor Add a copy...Ch. 8 - Carpet Calculator The Westfield Carpet Company has...Ch. 8 - LandTract Class Make a LandTract class that has...Ch. 8 - Month Class Write a class named Month. The class...Ch. 8 - CashRegister Class Write a CashRegister class that...Ch. 8 - Sales Receipt File Modify the program you wrote in...Ch. 8 - Parking Ticket Simulator For this assignment you...Ch. 8 - Geometry Calculator Design a Geometry class with...Ch. 8 - Car Instrument Simulator For this assignment, you...Ch. 8 - First to One Game This game is meant for two or...Ch. 8 - Heads or TaiLs Game This game is meant for two or...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Match the following terms to the appropriate definition: _____equi-join _____derived table _____natural join __...
Modern Database Management
How many "hello' output lines does this program print?
Computer Systems: A Programmer's Perspective (3rd Edition)
Essay Class Design an Essay class that extends the GradedActivity class presented in this chapter. The Essay cl...
Starting Out with Programming Logic and Design (4th Edition)
Use the following tables for your answers to questions 3.7 through 3.51 : PET_OWNER (OwnerID, OwnerLasst Name, ...
Database Concepts (7th Edition)
What is the type or types of the variable(s) that can reference the object created in the following statement? ...
Java: An Introduction to Problem Solving and Programming (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- solve with java Create a class for Subject containing the Name of the subject and score of the subject. There should be following methods: set: it will take two arguments name and score, and set the values of the attributes. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. set: it will take one double value as argument and set the value of score only. If score is less than 0 or greater than 100.0 then a message should be displayed “Incorrect score” and score should be set to Zero. display: it will display the name and score of the subject. Like “Name : Math, Score: 99.9” getScore: it will return the value of score. greaterThan: it will take subject’s object as argument, compare the calling object’s score with argument object’s score and return true if the calling object has greater score. Create a class “Main” having main method to perform following tasks. Create two objects of Subject class having…arrow_forwardjava programming language You are part of a team writing classes for the different game objects in a video game. You need to write classes for the two human objects warrior and politician. A warrior has the attributes name (of type String) and speed (of type int). Speed is a measure of how fast the warrior can run and fight. A politician has the attributes name (of type String) and diplomacy (of type int). Diplomacy is the ability to outwit an adversary without using force. From this description identify a superclass as well as two subclasses. Each of these three classes need to have a default constructor, a constructor with parameters for all the instance variables in that class (as well as any instance variables inherited from a superclass) accessor (get) and mutator (set) methods for all instance variables and a toString method. The toString method needs to return a string representation of the object. Also write a main method for each class in which that class is tested – create…arrow_forwardCode the StudentAccount class according to the class diagramMethods explanation:pay(double): doubleThis method is called when a student pays an amount towards outstanding fees. The balance isreduced by the amount received. The updated balance is returned by the method.addFees(double): doubleThis method is called to increase the balance by the amount received as a parameter. The updatedbalance is returned by the method.refund(): voidThis method is called when any monies owned to the student is paid out. This method can onlyrefund a student if the balance is negative. It displays the amount to be refunded and set thebalance to zero. (Use Math.abs(double) in your output message). If there is no refund, display anappropriate message.Test your StudentAccount class by creating objects and calling the methods addFees(), pay() andrefund(). Use toString() to display the object’s data after each method calledarrow_forward
- Library Information System Design and Testing Library Item Class Design and TestingDesign a class that holds the Library Item Information, with item name, author, publisher. Write appropriate accessor and mutator methods. Also, write a tester program(Console and GUI Program) that creates three instances/objects of the Library Items class. Extending Library Item Class Library and Book Classes: Extend the Library Item class in (1) with a Book class with data attributes for a book’s title, author, publisher and an additional attributes as number of pages, and a Boolean data attribute indicating whether there is both hard copy as well as eBook version of the book. Demonstrate Book Class in a Tester Program (Console and GUI Program) with an object of Book class.arrow_forwardRectangle Object Monitoring Create a Rectangle class that can compute the total area of all the created rectangle objects using static fields (variables). Remember that a Rectangle has two attributes: Length and Width. Implement the class by creating a computer program that will ask the user about three rectangle dimensions. The program should be able to display the total area of the three rectangle objects. For this exercise, you are required to apply all OOP concepts that you learned in class. Sample output: Enter Length R1: 1 Enter Width R1: 1 Enter Length R2: 2 Enter Width R2: 2 Enter Length R3: 3 Enter Width R3: 3 The total area of the rectangles is 14.00 Note: All characters in boldface are user inputs.arrow_forwardParking Ticket SimulatorFor this assignment you will design a set of classes that work together to simulate apolice officer issuing a parking ticket. The classes you should design are:• The Parkedcar Class: This class should simulate a parked car. The class's respon-sibilities are:To know the car's make, model, color, license number, and the number of min-utes that the car has been parkedThe BarkingMeter Class: This class should simulate a parking meter. The class'sonly responsibility is:- To know the number of minutes of parking time that has been purchased• The ParkingTicket Class: This class should simulate a parking ticket. The class'sresponsibilities areTo report the make, model, color, and license number of the illegally parked carTo report the amount of the fine, which is $2S for the first hour or part of anhour that the car is illegally parked, plus $10 for every additional hour or part ofan hour that the car is illegally parkedTo report the name and badge number of the police…arrow_forward
- Requirements A vehicle has a certain fuel efficiency (measured in miles/gallon) and a certain amount of fuel in the gas tank. We need to simulate driving the vehicle for a given distance while reducing the amount of gasoline in the fuel tank. We also need to be able to check how much gas is in the tank, and we need to be able to add gasoline to the fuel tank. Build the Vehicle class with the following specifications: Instance data:Variable mpg for fuel efficiency (miles per gallon = mpg)Variable gas to save how many gallons of gas left in the tank Constructors:Default constructor with no parameter. Use 0 as initial values.Overloaded constructor with two parameters Methods:getMPG() & setMPG()(getGas() & setGas()toString() methoddrive() to simulate that the car is driven for certain miles. For example, v1.drive(100) means vehicle v1 is driven 100 miles. You need to calculate the gas cost and update the gas tank: gas = gas - miles/mpg. You also need to check if there…arrow_forwardDefining Classes Write and document the definition for the Product class. Each Product object has three instance variables: description, productNumber and price. The class has one constructor that takes three parameters in the order productNumber, description and price. The class has one accessor method named getCost(int qty) which returns the price for qty items of the Product. The class has two mutator methods—setPrice() and setDescription()—that modify the corresponding instance variables. The class has a toString() method that returns the Product as a String in the form: 34567: Small Toaster, $ 17.95. Note: use concatenation to construct the returned string. Do not use the String.format() method to format the returned value.arrow_forwardRequirements A vehicle has a certain fuel efficiency (measured in miles/gallon) and a certain amount of fuel in the gas tank. We need to simulate driving the vehicle for a given distance while reducing the amount of gasoline in the fuel tank. We also need to be able to check how much gas is in the tank, and we need to be able to add gasoline to the fuel tank. Build the Vehicle class with the following specifications: Instance data:Variable mpg for fuel efficiency (miles per gallon = mpg)Variable gas to save how many gallons of gas left in the tank Constructors:Default constructor with no parameter. Use 0 as initial values.Overloaded constructor with two parameters Methods:getMPG() & setMPG()(getGas() & setGas()toString() methoddrive() to simulate that the car is driven for certain miles. For example, v1.drive(100) means vehicle v1 is driven 100 miles. You need to calculate the gas cost and update the gas tank: gas = gas - miles/mpg. You also need to check if there…arrow_forward
- Focus on classes, objects, methods and good programming style Your task is to create a BankAccount class. Class name BankAccount Attributes _balance float _pin integer Methods init () get_pin() check pin () deposit () withdraw () get_balance () The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The pin should be generated randomly when the account object is created. The initial balance should be 0. get_pin () should return the pin. check_pin (pin) should check the argument against the saved pin and return True if it matches, False if it does not. deposit (amount) should receive the amount as the argument, add the amount to the account and return the new balance. withraw (amount) should check if the amount can be withdrawn (not more than is in the account), If so, remove the argument amount from the account and return the new balance if the transaction was successful. Return False if it was not. get_balance () should return the current balance.…arrow_forwardTemp Class - Create Temp class whos job is to hold a temp in degrees F and gives methods to get the temp in F, Celsius, and Kelvin. The class should follow the instance variable (field): ftemp (double type that holds F temp)Follow these methods with the class.. Constructor --Will accept Fahrenheit temperature as a double to store it in the ftemp field. setFahrenheit - The setFahrenheit method accepts a Ftemperature as a doubleto stores it in the ftemp field. getFahrenheit - Returns the value of the ftemp field, as a Fahrenheit temperature (no conversion required). getCelsius - Returns the value of the ftemp field converted to Celsius. [C e l s i u s = ( 5.0 / 9.0 ) × ( F a h r e n h e i t − 32 )] getKelvin - Returns the value of the ftemp field converted to Kelvin. [K e l v i n = ( ( 5.0 / 9.0 ) × ( F a h r e n h e i t − 32 ) ) + 273] Exemplify the temp class by writing a (test) program that is separate and promotes users for an F Fahrenheit temp. The program must create an…arrow_forwardTrue or false: Just as a class can have multiple constructors, it can also have multiple destructors.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
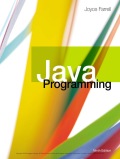
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY